Android
Android userland for bc9 (2/2)
Setting up Android userland 1 †
It is assumed that you get and build open source version of Android source code.
Before you proceed, please install and set up required applications and packages in advance to build Android source code.
Obtaining source †
- Make an directory:
$ mkdir mydroid
$ cd mydroid
- Init repo. This time we use master branch. (We are using source code checked out at March 20, 2009)
$ repo init -u git://android.git.kernel.org/platform/manifest.git
- Checkout source code.
$ repo sync
Make sure that following directories are exist:
$ ls
Makefile build external kernel system
bionic dalvik frameworks packages
bootable development hardware prebuilt
Adjustment for gumstix †
In order to run Android on gumstix, we add small modifications on some source codes.
(Some of these may be not necessary. Though we add these modification and succeeded.)
On April 2009 a lots of source code are merged from cupcake development branch to master branch. Due to this merging, further modifications may be required when you use current master branch source code.
(reference http://androidzaurus.seesaa.net/article/116995835.html )
Disable pmem
(reference http://groups.google.co.jp/group/android-embedded-japan/browse_thread/thread/bc549dab23f6cbac#)
pmem is a function to allocate a large continuous physical memory region.
When pmem is enabled, Android won't work on gumstix.
~/mydroid/system/core/init/devices.c
So modify this file as follows:
diff -u devices.c.original devices.c
--- devices.c.original 2009-03-30 19:05:50.000000000 +0900
+++ devices.c 2009-03-11 19:13:54.000000000 +0900
@@ -109,9 +109,9 @@
{ "/dev/eac", 0660, AID_ROOT, AID_AUDIO, 0 },
{ "/dev/cam", 0660, AID_ROOT, AID_CAMERA, 0 },
{ "/dev/pmem", 0660, AID_SYSTEM, AID_GRAPHICS, 0 },
- { "/dev/pmem_gpu", 0660, AID_SYSTEM, AID_GRAPHICS, 1 },
- { "/dev/pmem_adsp", 0660, AID_SYSTEM, AID_AUDIO, 1 },
- { "/dev/pmem_camera", 0660, AID_SYSTEM, AID_CAMERA, 1 },
+ { "/dev/pmem_gpu", 0660, AID_SYSTEM, AID_GRAPHICS, 0 },
+ { "/dev/pmem_adsp", 0660, AID_SYSTEM, AID_AUDIO, 0 },
+ { "/dev/pmem_camera", 0660, AID_SYSTEM, AID_CAMERA, 0 },
{ "/dev/oncrpc/", 0660, AID_ROOT, AID_SYSTEM, 1 },
{ "/dev/adsp/", 0660, AID_SYSTEM, AID_AUDIO, 1 },
{ "/dev/mt9t013", 0660, AID_SYSTEM, AID_SYSTEM, 0 },
Fixing drawing position of Boot animation
To fix position of Android's logo and robot blinking animation on start up, add following modifications.
(reference https://review.source.android.com/Gerrit#change,1552 )
- Modifying source code
~/mydroid/frameworks/base/libs/surfaceflinger/BootAnimation.cpp
Modify source code as follows:
diff -u BootAnimation.cpp.original BootAnimation.cpp
--- BootAnimation.cpp.original 2009-03-30 19:53:25.000000000 +0900
+++ BootAnimation.cpp 2009-03-25 20:59:04.000000000 +0900
@@ -196,6 +196,7 @@
return r;
}
+static const int RIGHT_MARGIN = 112;
bool BootAnimation::android()
{
- initTexture(&mAndroid[0], mAssets, "images/android_320x480.png");
+ initTexture(&mAndroid[0], mAssets, "images/android_480x272.png");
initTexture(&mAndroid[1], mAssets, "images/boot_robot.png");
initTexture(&mAndroid[2], mAssets, "images/boot_robot_glow.png");
@@ -219,24 +221,28 @@
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
glTexEnvx(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE);
const int steps = 8;
+ int x = (mWidth - mAndroid[0].w)/2;
+ int y = (mHeight - mAndroid[0].h)/2;
+ x = (x < 0) ? 0 : x;
+ y = (y < 0) ? 0 : y;
for (int i=1 ; i<steps ; i++) {
float fade = i / float(steps);
glColor4f(1, 1, 1, fade*fade);
glClear(GL_COLOR_BUFFER_BIT);
- glDrawTexiOES(0, 0, 0, mAndroid[0].w, mAndroid[0].h);
+ glDrawTexiOES(x, y, 0, mAndroid[0].w, mAndroid[0].h);
eglSwapBuffers(mDisplay, mSurface);
}
// draw last frame
glTexEnvx(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_REPLACE);
glDisable(GL_BLEND);
- glDrawTexiOES(0, 0, 0, mAndroid[0].w, mAndroid[0].h);
+ glDrawTexiOES(x, y, 0, mAndroid[0].w, mAndroid[0].h);
eglSwapBuffers(mDisplay, mSurface);
// update rect for the robot
- const int x = mWidth - mAndroid[1].w - 33;
- const int y = (mHeight - mAndroid[1].h)/2 - 1;
+ x = mWidth - (mWidth - mAndroid[0].w)/2 - mAndroid[1].w - RIGHT_MARGIN;
+ y = (mHeight - mAndroid[1].h)/2 - 1;
const Rect updateRect(x, y, x+mAndroid[1].w, y+mAndroid[1].h);
// draw and update only what we need
- Replace image file
From the image file used in BootAnimation.cpp,
~/mydroid/frameworks/base/core/res/assets/images/android_320x480.png
We made an image file android_480x272.png, fitted to gumstix LCD's resolution.
Put this android_480x272.png file in same directory as android_320x480.png.
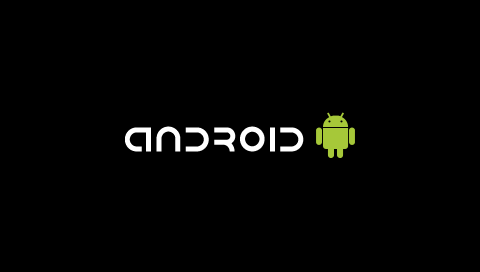
Modifying key event check
(reference http://ozetchi.cocolog-nifty.com/amerika/2009/02/post-6a82.html )
~mydroid/frameworks/base/services/java/com/android/server/KeyInputQueue.java
Modify this file as follows:
diff -u KeyInputQueue.java.original KeyInputQueue.java
--- KeyInputQueue.java.original 2009-03-30 19:23:51.000000000 +0900
+++ KeyInputQueue.java 2009-03-11 12:31:56.000000000 +0900
@@ -247,7 +247,7 @@
}
if (!send) {
- continue;
+// continue;
}
synchronized (mFirst) {
This modification is for accepting key input event certainly.
build †
After you finish those modifications, move to mydroid directory and build:
$ cd ~/mydroid
$ make
Android userland for bc9 (2/2)
by Satoshi OTSUKA
|